In our previous article, we discussed how to Get API Requests using Playwright. Now, Let's create a POST request using Playwright.
Table of Contents
Demo API
For the demo, let's use Create user POST API of the reqres website.
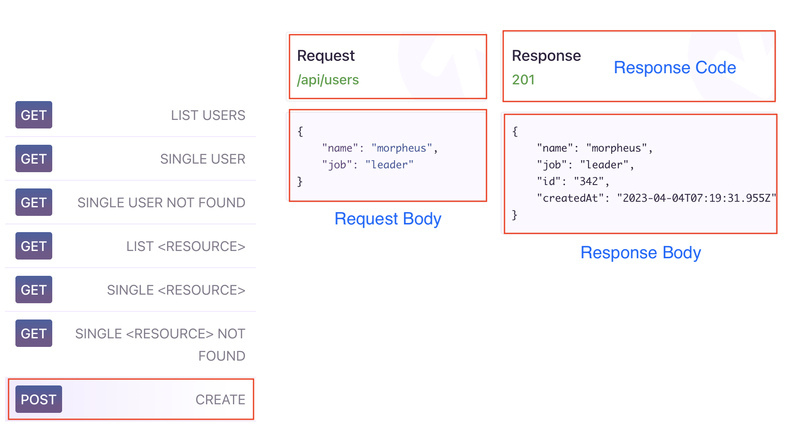
How to Create Post Request
Step 1: Use Request Instance as Parameter
test.only('Post Request', async({request}) =>{
})
Step 2: Create HTTP Post Request and store response in a variable
const response = await request.post("https://reqres.in/api/users")
Add request value highlighted in screenshort to URL of the website.
Step 3: Add Request Body to Post Method
const response = await request.post("https://reqres.in/api/users",{
data: {
"name": "morpheus",
"job": "leader"
}
})
Step 4: Verify Response code is 201
expect(response.status()).toBe(201)
If you want to print the response in the console, use the code below.
console.log(await response.json())
Code:
test.only('Post Request', async({request}) =>{
const response = await request.post("https://reqres.in/api/users",{
data: {
"name": "morpheus",
"job": "leader"
}
})
expect(response.status()).toBe(201)
console.log(await response.json())
})
- Log in to post comments