We all have heard about inheritance. Well, that's the topic we will discuss today in cypress on how to inherit contents by sharing classes.
This is a crucial topic in cypress as it is used well in its framework. Let us create classes in cypress and understand how inheritance works, or we can say abstraction with classes too.
For example, we will be automating our programsbuzz login site. This tutorial will be solely based on that.
1.) BasePage Class.
Create a class name BasePage and create a static method called visitPage this class is designed mainly to visit the URL.
The file should be a BasePage.js file.
class BasePage{
static visitPage() {
cy.visit("http://www.programsbuzz.com/user/login");
}
}
export default BasePage
Make sure to export BasePage, in the end to import it in other classes and tests.
2.) Home Class.
Let us create a Home class js file.
import BasePage from "./BasePage";
class Home extends BasePage{
static userName() {
cy.get("#edit-name").type('Naruto');
}
static passWord() {
cy.get("#edit-pass").type('Rasengan');
}
}
export default Home
In this BasePage will be extended and imported as well.
Here two methods are created userName and passWord.
3.)Spec File.
Let us create a normal spec file and import the Home class.
import Home from "./Home";
describe('Abstract',()=>{
it('Login',()=>{
Home.visitPage();
Home.userName();
Home.passWord();
})
})
We can witness here that after importing the Home class, we can access the contents from BasePage and those inherited by the child class Home.
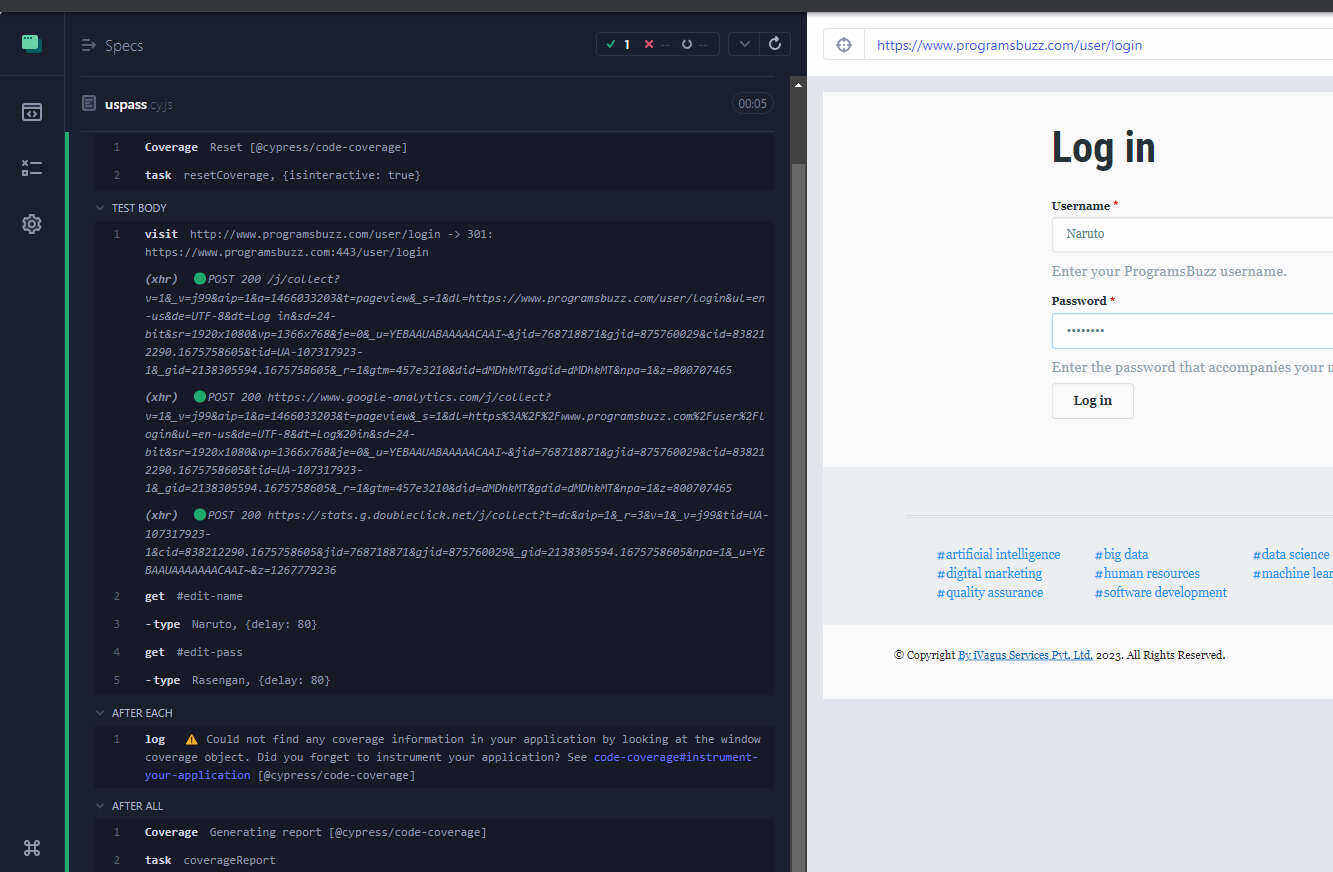
Test Hooks:
Hooks can also be implemented while abstracting classes.
import Home from "./Home";
describe('Abstract',()=>{
before(function () {
Home.visitPage();
});
it('Login',()=>{
Home.userName();
Home.passWord();
})
})
Before hook is used here to run the test before all test step methods.
Likewise, other hooks such as after(), beforeEach(), and afterEach() can also be implemented.
Skip And Only.
Likewise, skip and only can also be implemented in this.
import Home from "./Home";
describe('Abstract',()=>{
before(function () {
Home.visitPage();
});
it.skip('Login With Correct Credentials',()=>{
Home.userName();
Home.passWord();
})
it.only('Login With Incorrect Credentials',()=>{
Home.userName();
Home.passWord();
})
})
Here skip and only is used on these two same test steps, which have two different credentials input.
- Log in to post comments