In Java, there is a wrapper class that wraps or, we can call, encapsulates primitive datatype into an object.
They are converted in such a way that when there arises a situation for a need of objects such as collections and generic types or other object-oriented constructs.
Eight wrapper classes in Java correspond to the eight primitive data types:
Primitive | Wrapper |
int | Integer |
long | Long |
short | Short |
byte | Byte |
double | Double |
float | Float |
char | Char |
boolean | Boolean |
Why wrapper class?
- The wrapper class is worthy because of its ability to convert primitive data types into objects.
- Objects are useful when it is needed for the arguments to be modified.
- Java.util classes only handle objects, so the wrapper class here is helpful.
- The object is crucial for multithreading.
public static void main(String[] args) {
int a = 100;
Integer intWr = new Integer(a);
System.out.println(intWr);
long b = 84848484;
Long strWr = new Long(b);
System.out.println(b);
}
- Here we used the wrapper class to convert primitive value int and long to objects Long and Int.
- Although this method approach is deprecated instead, we can use this.
public static void main(String[] args) {
int a = 100;
Integer intWr = Integer.valueOf(a);
System.out.println(intWr);
long b = 84848484;
Long strWr = Long.valueOf(b);
System.out.println(b);
}
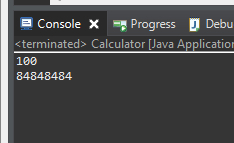
- Log in to post comments