StringBuilder is a class used in Java to manipulate and operate on strings. Unlike regular strings, which cannot be changed or modified.
Objects of StringBuilder can be modified and updated without having to create a new object every time.
StringBuilder s = new StringBuilder("Naruto");
- StringBuilder object created with the initial value.
- The StringBuilder class has various methods for adding and appending values to the string, including append(), insert(), and replace():
s.append(" Uzumaki");
sb.insert(6, ",");
sb.replace(0, 3, "ka");
- Append: Add the Uzumaki word next to the initial value.
Insert: inserts comma at the 6th index.
Replace: replaces the first three characters with "ka."
The StringBuilder class also has methods for deleting and removing characters from the string, such as delete() and deleteCharAt():
s.delete(0, 4);
s.deleteCharAt(sb.length() - 2);
- Delete: Deletes the first three characters.
deleteCharAt: Deletes last character.
Other methods in stringbuilder such as length(), charAt(), toString() also comes handy:
int length = s.length();
char c = s.charAt(0);
String str = s.toString();
length: Returns the length of the string
charAt: Returns the first character of the string
toString: Converts the StringBuilder to a regular string
package week4.day2;
public class boom {
public static void main(String[] args) {
StringBuilder s = new StringBuilder("Naruto");
s.append(" Uzumaki");
s.insert(2, ",");
s.replace(0, 2, "ka");
s.delete(0, 5);
s.deleteCharAt(s.length() - 3);
int length = s.length();
char charAt = s.charAt(1);
String string = s.toString();
System.out.println(s);
System.out.println(length);
System.out.println(charAt);
System.out.println(string);
}
}
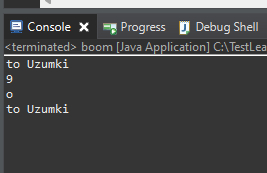
Conclusion:
Overall, the StringBuilder class is a valuable tool for creating and manipulating strings in Java, especially when dealing with large amounts of text that must be modified frequently.
- Log in to post comments