To select a checkbox in a webpage using selenium, we must first locate the checkbox using one of the locating mechanisms.
Either id, name do this, or even class name or xpath is handy. Simply using the click() method, we can select a checkbox.
This is the site we are going to be selecting the checkbox on.
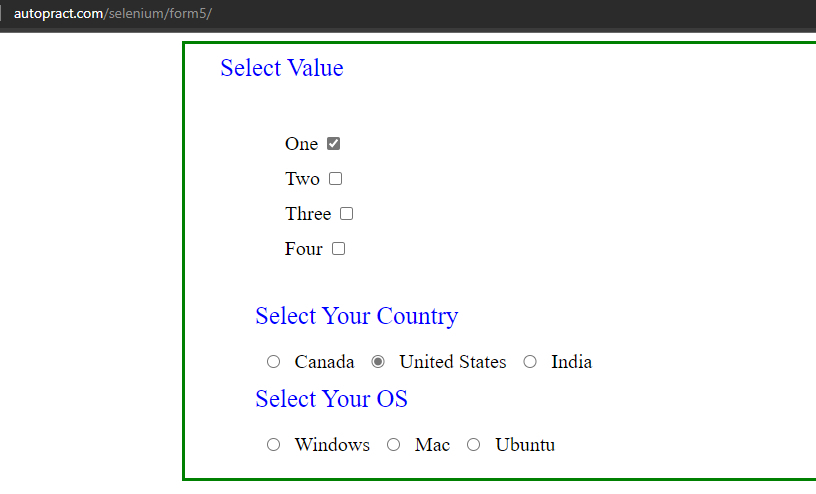
Let us select three checkbox first using xpath by its value.

WebDriverManager.chromedriver().setup();
ChromeDriver driver = new ChromeDriver();
driver.get("http://autopract.com/selenium/form5/");
driver.manage().window().maximize();
driver.findElement(By.xpath("//input[@value='three']")).click();
- Doing this will click the three checkbox.
Let us make a more dynamic approach to click the checkbox dynamically.
{
WebDriverManager.chromedriver().setup();
ChromeDriver driver = new ChromeDriver();
driver.get("http://autopract.com/selenium/form5/");
driver.manage().window().maximize();
List<WebElement> checkBoxes = driver.findElements(By.xpath("//label[@class='container']"));
for (WebElement cB : checkBoxes) {
String cBTexts = cB.getText();
System.out.println(cBTexts);
if (cBTexts.equalsIgnoreCase("Four")) {
cB.click();
}
}
}
}
- Here we took the list of checkboxes and iterated it in the advanced loop.
- Then we wrote a condition if the text is equal to the text we provided, then clicked on it.
- Log in to post comments