In Playwright, you can get the element's text using the textContent() method or a set of elements using the allTextContents() method.
Table of Content
- Syntax
- Demo Website
- Get Text of an Element
- Get First Element Text from the List
- Get Last Element Text from the List
- Get Nth Element Text from the List
- Get All Elements Text from the List
- Iterate and Compare Text
- Video Tutorial
1. Syntax
- page.textContent(selector[, options]) - In options we can specify strict and timeout.
- locator.textContent([options]) - In options you we can specify timeout.
- locator.allTextContents()
We can also use textContent() method with frame and elementHandle.
2. Demo Website
For a single element, let's get the text Get An Answer To Your Technical Queries from the homepage's banner, and for a list of elements, let's use the header menu of the programsbuzz website.
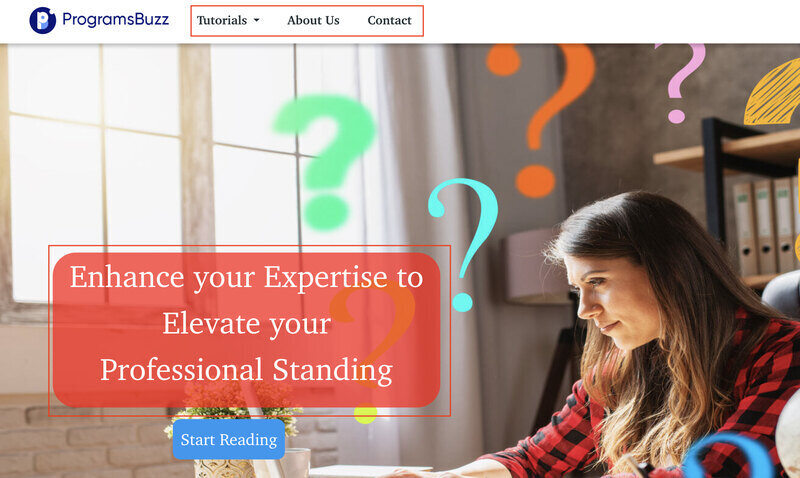
3. Get Text of an Element
Using locator method
When single elements return, we can use the textContent() method with the locator.
// Print Text - Enhance your Expertise to Elevate your Professional Standing
console.log(await page.locator('div#slide-7-layer-1').textContent())
Using page Fixture
We can also use the textContent() method with the page fixture.
// Print Text - Enhance your Expertise to Elevate your Professional Standing
console.log(await page.textContent('div#slide-7-layer-1'));
4. Get First Element Text from the List
Using locator method
The locator method will return a strict mode violation error because selector will resolved to 5 elements.
// Strict Mode Violation Error
await page.locator('ul.we-mega-menu-ul>li>a').textContent();
Here, we can use the first() method, nth() method, nth selector, Xpath, and CSS selector or any other technique. Let's use the first() method.
// Print Text - Tutorials
console.log(await page.locator('ul.we-mega-menu-ul>li>a').first().textContent());
Using page Fixture
When multiple elements return, the page fixture will return the first element by default.
// Print Text - Tutorials
console.log(await page.textContent('ul.we-mega-menu-ul>li>a'));
However, if you will set the strict option to true, it will return a strict mode violation error.
// Strict Mode Violation Error
await page.textContent("ul.we-mega-menu-ul>li>a",{strict:true});
When strict mode is on, we can use the nth selector, XPath selector, or CSS selector to get the text of the first element. We can not use the nth(), first(), and last() methods with the page fixture. Let's use the nth selector with index 0 to get the first element.
// Returns Text - Tutorials
await page.textContent("ul.we-mega-menu-ul>li>a>>nth=0",{strict:true});
5. Get Last Element Text from the List
Using locator method
Similarly, to avoid strict mode violation in case of multiple elements, we can use the last() method, nth() method, nth selector, CSS selector, or XPath selector. Let's use the last() method.
// Print Text - Contact
console.log(await page.locator('ul.we-mega-menu-ul>li>a').last().textContent());
Using page Fixture
We can use the nth selector, CSS selector, or XPath selector with page fixture. Let's use the nth selector and index -1 to get the content of the last element.
// Print Text - Contact
console.log(await page.textContent("ul.we-mega-menu-ul>li>a>>nth=-1",{strict:true}));
6. Get Nth Element Text from the List
Using locator method
We can use the nth() method, nth selector, XPath, or CSS selector to get a text from the nth element. Let's get the text of the 2nd element using the nth() method and index 1.
// Print Text - About Us
console.log(await page.locator('ul.we-mega-menu-ul>li>a').nth(1).textContent());
Using page Fixture
We can use the nth selector, XPath, or CSS selector to get a text from the nth element. Let's get the text of the 2nd element using the nth selector and index 1.
// Returns Text - Interview Questions
console.log(await page.textContent("ul.we-mega-menu-ul>li>a>>nth=1",{strict:true}));
7. Get All Elements Text from the List
With the locator method, you can use the allTextContent() method, which returns an array of textContent values for all matching nodes.
console.log(await page.locator('ul.we-mega-menu-ul>li>a').allTextContents());
It will return text of all 3 elements in header
[
'\n Tutorials ',
'\n About Us ',
'\n Contact '
]
7. Iterate and Compare Text
You can iterate through each value of the array using a loop.
const mylist = await page.locator('ul.we-mega-menu-ul>li>a').allTextContents();
for (let i = 0; i < mylist.length;i++)
{
if(mylist[i].trim()==='About Us')
{
console.log("element found in list")
break;
}
}
The Loop will break after finding About Us in the list.
- Log in to post comments