The set extends the Collection interface and is included in Java.util package. It is an unordered collection of objects that cannot store duplicate values. It is a mathematical set implementation interface. This interface inherits the Collection interface's methods and adds a feature preventing identical elements' insertion. SortedSet and NavigableSet are two interfaces that expand the set implementation.
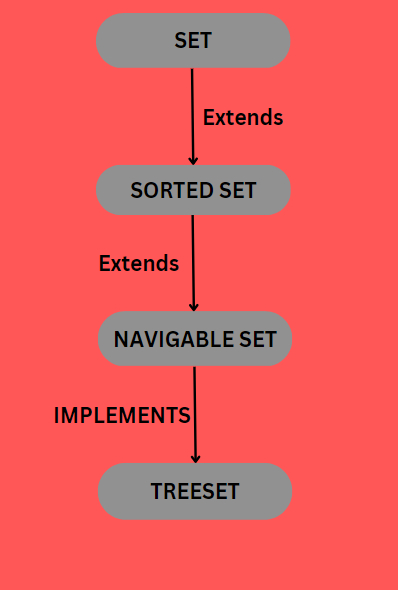
- The navigable set interface extends the sorted set interface in the illustration above.
- Because a set does not keep its insertion order, the navigable set interface provides an implementation for traversing the Set.
- The navigable Set is implemented by a TreeSet, which implements a self-balancing tree. As a result, this interface allows us to traverse across this tree.
Example:
package week1.day2;
import java.util.LinkedHashSet;
import java.util.Set;
public class Calculator {
public static void main(String[] args) {
Set<String> showName = new LinkedHashSet<String>();
showName.add("One");
showName.add("Piece");
System.out.println(showName);
}
}
- Here is an example of a Set.
- We created a linked HashSet using a set.
Output:
[One, Piece]
Set Interface Operation
We can do all basic mathematical operations on the Set, such as intersection, union, and difference.
Consider the following two sets: set1 = [2,5,3,7,8] and set2 = [2,7,4,9,1]. On the Set, we can do the subsequent operation:
- Intersection: The intersection operation returns all elements that appear in both sets. Set1 and set2 will intersect at [2,7].
- Union: The union operation returns all elements of sets 1 and 2 in a single set, which can be set1 or set2. The sum of sets 1 and 2 is [1,2,3,4,5,7,8,9].
- Difference: The difference operation removes any values present in another set from the set. The difference between sets 1 and 2 is [5,3,8,4,9,1].
- Log in to post comments