Capturing network traffic with Selenium WebDriver in Java necessitates using extra tools and libraries in addition to the core WebDriver capabilities. One typical solution is to employ a proxy server to intercept and analyze the WebDriver's network queries. BrowserMob Proxy is a popular proxy server for this purpose.
To collect network traffic in Java using Selenium WebDriver, follow these steps:
Set up the required dependencies:
The BrowserMob Proxy library (JAR file) can be obtained from the official website or the Maven repository.
<dependency>
<groupId>net.lightbody.bmp</groupId>
<artifactId>browsermob-core</artifactId>
<version>2.1.5</version>
<scope>test</scope>
</dependency>
Add the BrowserMob Proxy JAR file to the build path of your project or dependency management tool (e.g., Maven or Gradle).
Configure the BrowserMob Proxy server to gather network traffic and start it:
BrowserMobProxy server = new BrowserMobProxyServer();
server.start(0);
Set up the WebDriver to use the proxy server as follows:
Proxy seleniumProxy = ClientUtil.createSeleniumProxy(server);
DesiredCapabilities capabilities = new DesiredCapabilities();
capabilities.setCapability(CapabilityType.PROXY, seleniumProxy);
capabilities.acceptInsecureCerts();
capabilities.setCapability(CapabilityType.ACCEPT_INSECURE_CERTS, true);
EnumSet <CaptureType> captureTypes = CaptureType.getAllContentCaptureTypes();
captureTypes.addAll(CaptureType.getCookieCaptureTypes());
captureTypes.addAll(CaptureType.getHeaderCaptureTypes());
captureTypes.addAll(CaptureType.getRequestCaptureTypes());
captureTypes.addAll(CaptureType.getResponseCaptureTypes());
server.setHarCaptureTypes(captureTypes);
server.newHar("MyHar");
System.setProperty("webdriver.chrome.driver","C:\\Users\\arili\\eclipse-workspace\\mytesting\\target\\chromedriver.exe");
ChromeOptions options = new ChromeOptions();
options.merge(capabilities);
WebDriver driver = new ChromeDriver(options);
We have adjusted the capture types and passed the capabilities to the driver.
Retrieve the network traffic data that was captured:
driver.get("https://www.programsbuzz.com");
Har har = server.getHar();
File myHarFile = new File("C:\\Users\\arili\\eclipse-workspace\\mytesting\\target\\PB.har");
har.writeTo(myHarFile);
System.out.println("HAR details successfully written...");
Here we can create a file to write on, and the har file will be made with the necessary traffic logs of the network.
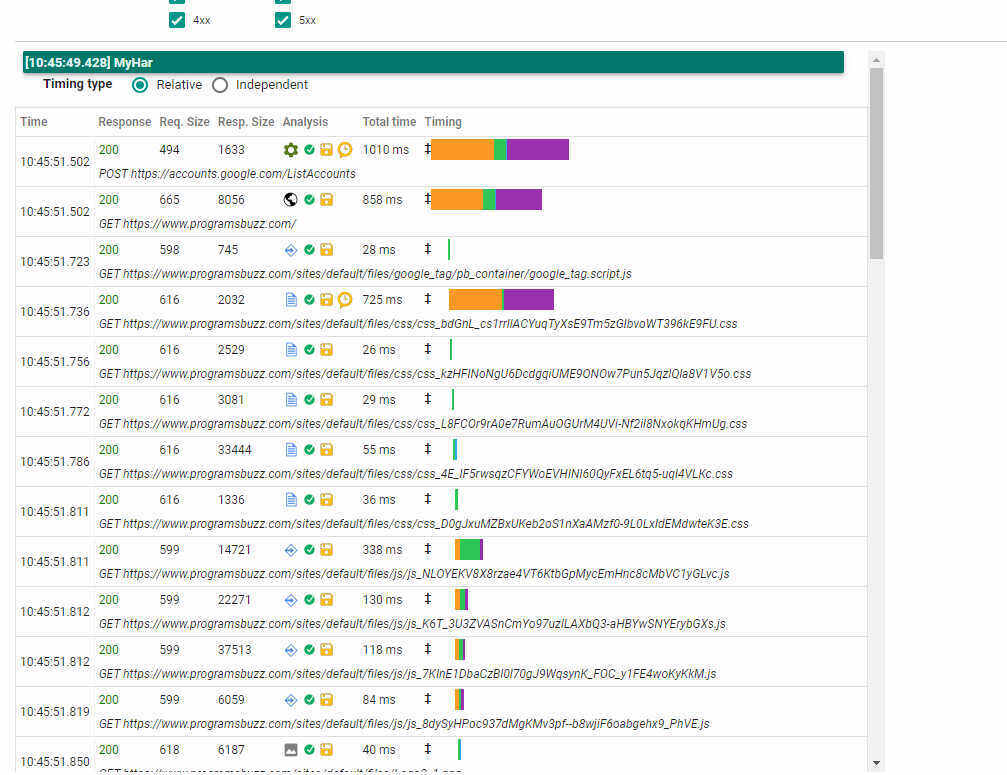
Here is the har file that was generated. We used an online har file viewer to view the har file.
- Log in to post comments