Autocomplete Now, what is it? When we type in the google or amazon search box, we get a bunch of pair suggestions according to our search terms.
Now, these are called autocomplete or autosuggestion boxes.
Let us see how we can handle it. We will be using amazon and makemytrip, for example.
1.) Amazon
Let us type iPhone in the search box of amazon.in
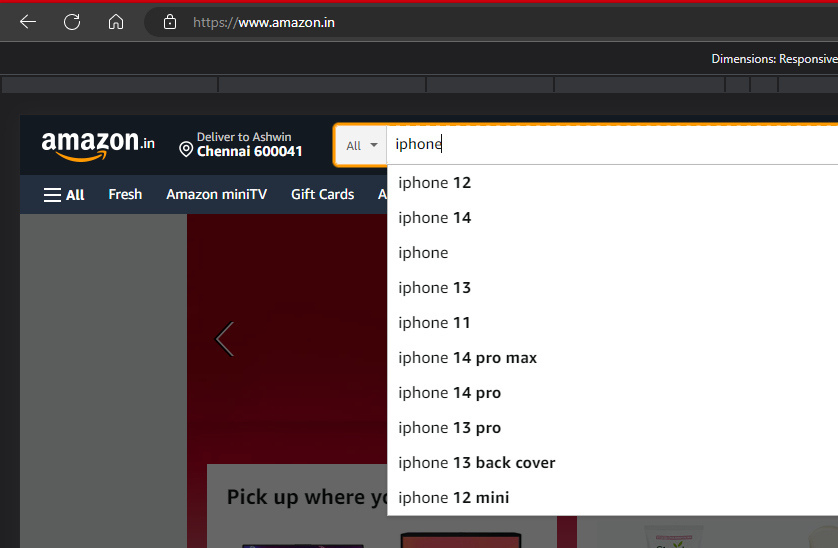
Let's select the iPhone 14 pro max from this list of suggestions.
WebDriverManager.chromedriver().setup();
ChromeDriver driver = new ChromeDriver();
driver.get("http://www.amazon.in");
driver.manage().window().maximize();
driver.findElement(By.id("twotabsearchtextbox")).sendKeys("iphone");
Thread.sleep(5000);
List<WebElement> searchListAutoComplete = driver
.findElements(By.xpath("//*[@id='nav-flyout-searchAjax']/div[2]"));
for (WebElement ele : searchListAutoComplete) {
String searchTexts = ele.getText();
System.out.println(searchTexts);
if (searchTexts.contains("iphone 14 pro max")) {
ele.click();
}
}
- After we sendkeys to the search bar, we take out the list of elements that are showing below and iterate it using the advanced for a loop.
- Doing this will yield an ele object with which we can get the texts of the suggestions that are coming below.
- Then using a simple if statement, we can click on the iPhone 14 pro max using contains method.
2.) MakeMyTrip
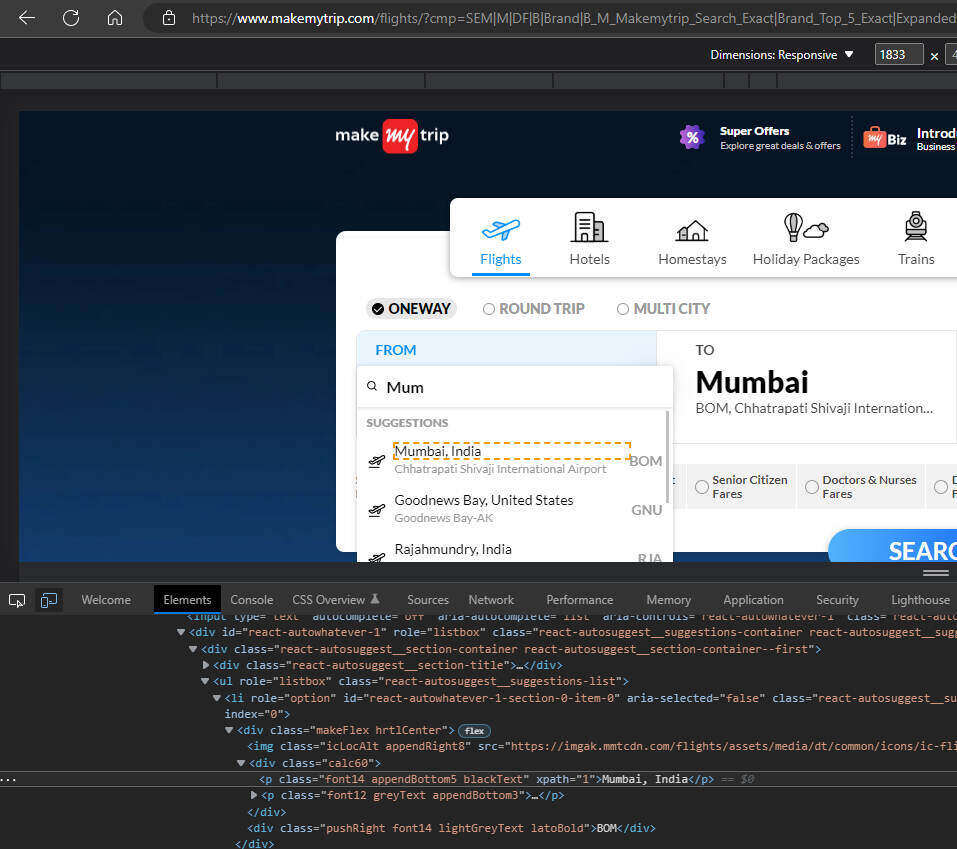
- Here from the pic, we can see that after typing in the city name; we can see dropdowns suggestion that let us handle the same way we did with amazon.
WebDriverManager.chromedriver().setup();
ChromeDriver driver = new ChromeDriver();
driver.get("http://www.makemytrip.com");
driver.manage().window().maximize();
String city = "Mumbai, India";
driver.findElement(By.xpath("//input[@id='fromCity']")).click();
driver.findElement(By.xpath("//input[@placeholder='From']")).sendKeys("Mum");
Thread.sleep(5000);
List<WebElement> cities = driver.findElements(By.xpath("//ul[@role='listbox']"));
for (WebElement ele : cities) {
String citiesText = ele.getText();
System.out.println(citiesText);
if (citiesText.contains(city)) {
ele.click();
}
}
- After getting the list of cities the same way as before, we iterated using the advanced loop, conditioned with an if statement, and clicked on the town.
- Log in to post comments