Frames in Selenium refer to HTML frames, which allow multiple HTML pages to be displayed on a single web page. In Selenium, you can switch between frames using the following methods:
- switchTo().frame(index) - switch to the frame at the specified zero-based index
- switchTo().frame(name or id) - switch to the frame with the specified name or ID
- switchTo().frame(WebElement) - switch to the frame containing the specified WebElement
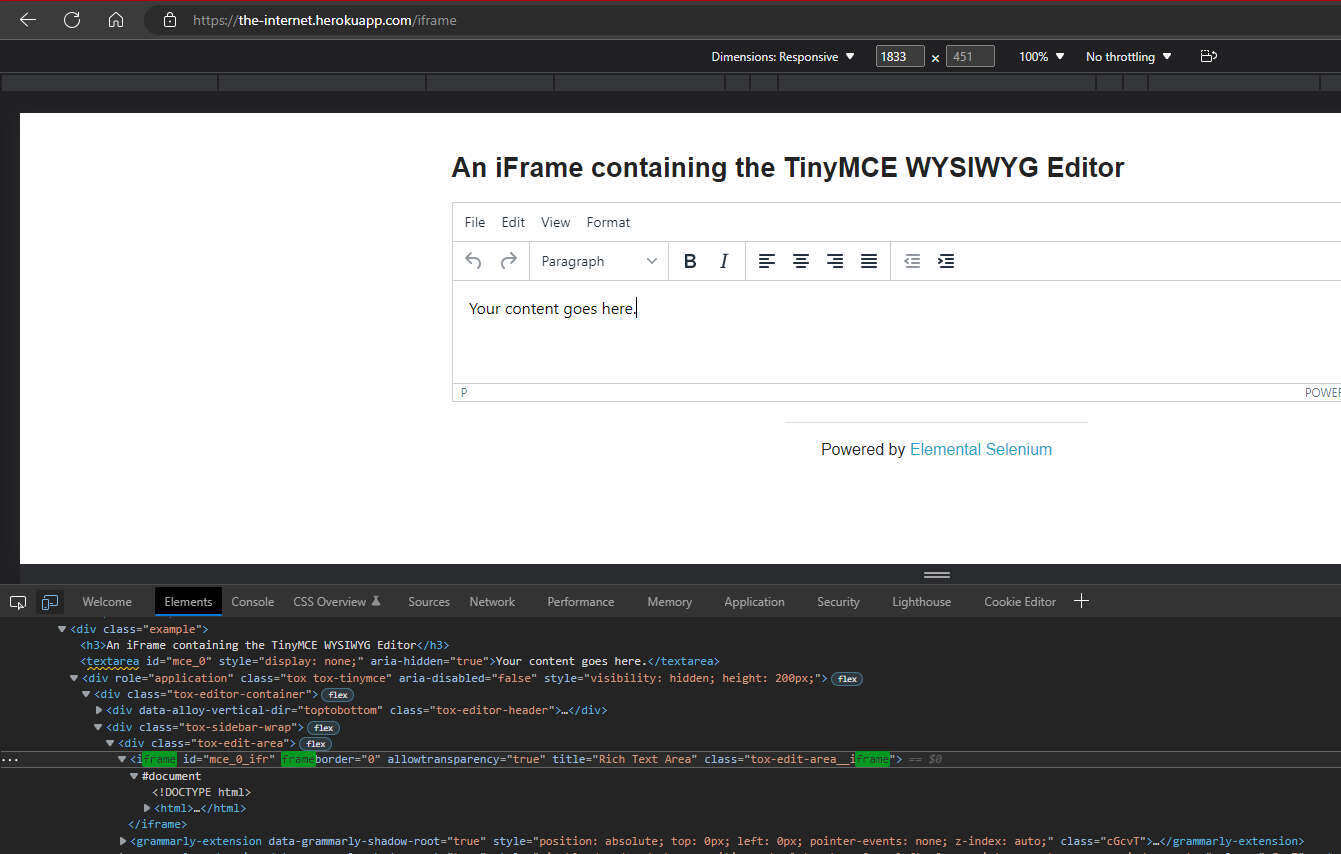
- This is the site we will use to play around with frames.
Index:
- Let us first try to switch based on the index.
WebDriverManager.chromedriver().setup();
ChromeDriver driver = new ChromeDriver();
driver.get("https://the-internet.herokuapp.com/iframe");
driver.manage().window().maximize();
WebDriver frameEntry = driver.switchTo().frame(0);
WebElement keysSend = frameEntry.findElement(By.id("tinymce"));
keysSend.sendKeys("Ashwin is here");
- As the frame from this site is in the zeroth index, we can use zero.
WebDriver frameEntry = driver.switchTo().frame(0);
Name/ID:
- Let us try to switch using name or id.
WebDriver frameEntry = driver.switchTo().frame("mce_0_ifr");
- Here we have used "id" and did the same operation webdriver successfully identify the frame.
WebElement:
- Let us try to use webelement to locate the frame and switch to it.
WebDriverManager.chromedriver().setup();
ChromeDriver driver = new ChromeDriver();
driver.get("https://the-internet.herokuapp.com/iframe");
driver.manage().window().maximize();
WebElement frame = driver.findElement(By.xpath("(//iframe[@id='mce_0_ifr'])[1]"));
WebDriver frameEntry = driver.switchTo().frame(frame);
WebElement keysSend = frameEntry.findElement(By.id("tinymce"));
keysSend.sendKeys("Ashwin is here");
- Here we used xpath for the frame, stored it in a variable, and passed it into the switchto frame.
Conclusion:
That is much about frames, and if needed to go back outside the frame, use this.
driver.switchTo().defaultContent();
- Log in to post comments