In this article, we will see what expected exceptions are in TestNG. TestNG provides the option to trace exceptions while handling code.
We can test codes to see whether it throws the desired exception. expectedExceptions parameter is used along with the @Test annotation, and now let us see that in action.
TestNG allows us to throw this exception we anticipate and ignore it, or we can call make it an exception.
Syntax:
@Test ( expectedExceptions = { IOException.class, NullPointerException.class } )
Example:
package week4.day2;
import org.testng.annotations.Test;
public class Ex {
@Test
private void test1() {
System.out.println("First");
}
@Test
private void test2() {
System.out.println("Second");
}
@Test
private void test3() {
int a = 0;
int b = 4;
System.out.println(b / a);
System.out.println("Third");
}
@Test
private void test4() {
System.out.println("Four");
}
}
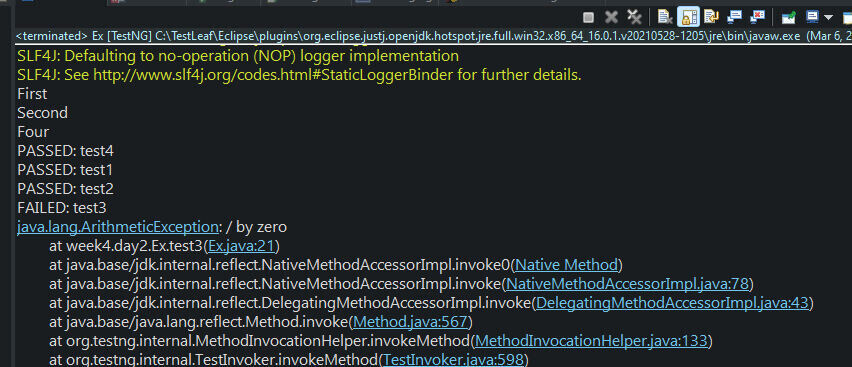
- When run, we see here in the test3 we are getting an arithmetic exception.
- Let us handle this using the expected exception.
@Test(expectedExceptions = ArithmeticException.class)
private void test3() {
int a = 0;
int b = 4;
System.out.println(b / a);
System.out.println("Third");
}
- Now we know that we will be expecting an arithmetic exception. Here we are telling testng via expected exception handling that let me pass.
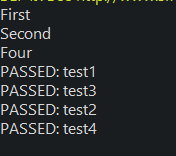
- We can see here that the test has passed, the exception has been ignored, and the other tests have run.
- Log in to post comments