Before
So in cypress, we have the hook called before where we can reach some test functionalities even before the test starts. This makes the test faster as the load gets executed before the test step.
- Let us say we have multiple numbers of tests in a single spec file and in every test we have to open a page and test its integrity so we cannot rely on .visit every time.
- So before hook comes into play which will open up the page before the load each and whenever we declare something inside this before hook block.
- Note that when we use before hook it only gets loaded for the first test step alone and its state of the browser gets cleared after a test step meaning before can only be utilized once per test step.
So before we go into the code, we are going to automate three things in http://www.autopract.com/#/home/fashion.
Step 1:- We need to visit the page.
Step 2:- We are going to close the appearing popup.
Step 3:- We are going to click the side tab.
Step 4:- We will assert whether the two fashion items, footwear, and watches are visible or not.
Sounds fun, right? Let us dive in.
describe('Automate AutoPract',()=>{
before(() => {
cy.visit('http://www.autopract.com/#/home/fashion')
cy.get('.close').as('Popup')
})
it('Should click POPUP',()=>{
cy.get('@Popup').click()
})
it('Verify Footwear Fashion',()=>{
cy.get('.bar-style').click()
cy.get('a').contains(' footwear ').should('be.visible')
})
it('Verify Watches Fashion', ()=> {
cy.get('a').contains(' watches ').should('be.visible')
})
})
- So here, we have implemented the following test steps ]with before hook.
- Now, why did we use before hook to visit the page? Why not via the test step itself?
- As we discussed earlier, using the test step rather than before the hook to visit the page takes a slight delay.
- If we use it before hook like this, we can load it fast enough that cypress will finish the tests earlier than usual within a snap.
So as you can see, it works flawlessly.
After
- There is a hook called "after" in cypress where we can fix this hook to run only once after all test cases have finished.
- This hook is used for cleaning up the state of our application after each test.
- After hook is also for asserting what is happening during the test.
- This is also used to delete any data created by a test or to ensure that a specific element is no longer visible on the page.
Let us take the same example as before hook and automate autopract site.
describe('Automate AutoPract',()=>{
before(() => {
cy.visit('http://www.autopract.com/#/home/fashion')
cy.get('.close').as('Popup')
})
it('Should click POPUP',()=>{
cy.get('@Popup').click()
})
after(function(){
cy.log('Test Ended')
})
})
This should finish the test and print the log inside after the hook.
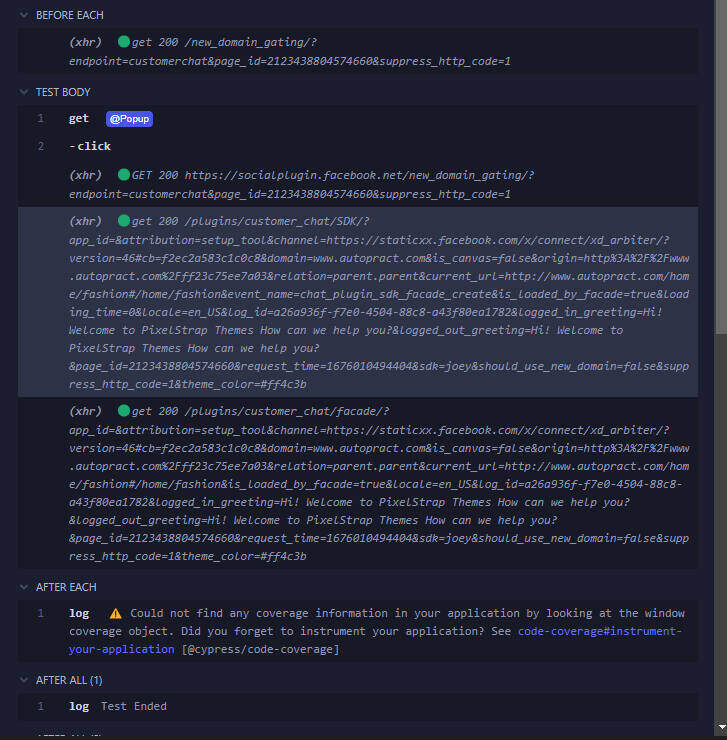
We can see after all hooks or after the hook has been triggered after the test is completed successfully.
- Log in to post comments