This article will show how we can work with the BDD framework in selenium. Behavior-Driven Development (BDD) is an agile software development approach emphasizing collaboration between developers, testers, and business stakeholders.
BDD frameworks are used to automate software testing using the BDD approach. BDD can be blended with selenium, an automation tool for functional testing.
Why BDD?
- Cucumber is a popular BDD framework that allows you to write test scenarios in natural language. Cucumber supports multiple programming languages, including Java, Ruby, and JavaScript.
- Using cucumber can be easy to read and understand the application flow.
- It acts as the bridge between technical and non-technical people.
- It benefits the client to understand the code easily using gherkin.
1.) Installation
<!-- https://mvnrepository.com/artifact/io.cucumber/cucumber-java -->
<dependency>
<groupId>io.cucumber</groupId>
<artifactId>cucumber-java</artifactId>
<version>7.11.1</version>
</dependency>
Use this dependency to install cucumber onto the already current selenium maven project.
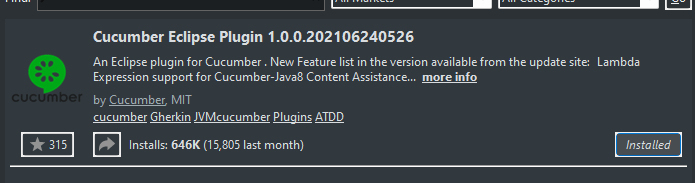
- Inside the eclipse marketplace, we need to install this cucumber eclipse plugin.
- After this Installation is complete.
2.) LoginPage Example
Feature:
Let us create a new feature file inside our project.

Let us create a new feature file with the same name as the extension in a new folder.
Feature: login page of Application
Scenario: Verification of a login
Given Open the chrome and launch the Application
Then Enter the Username and Password
The feature file is about opening and logging the application login page we will test.
Runner Class:
package TestRunner;
import org.junit.runner.RunWith;
import io.cucumber.junit.Cucumber;
import io.cucumber.junit.CucumberOptions;
@RunWith(Cucumber.class)
@CucumberOptions(features = "C:\\Users\\arili\\git\\Assignments\\Selenium\\feature\\Myfeature.feature", glue = {
"StepDefinition" })
public class Runner {
}
- Create a runner class separately in our project.
@RunWith() annotation will start executing our tests.
@CucmberOptions() annotation sets options like feature file, step definition, etc.
Step Definition:
After implementing the missing steps from the step definition.
package StepDefinition;
import org.openqa.selenium.By;
import org.openqa.selenium.chrome.ChromeDriver;
import io.cucumber.java.en.Given;
import io.cucumber.java.en.Then;
import io.github.bonigarcia.wdm.WebDriverManager;
public class Steps {
ChromeDriver driver;
@Given("Open the chrome and launch the application")
public void Open_the_chrome_and_launch_the_() {
WebDriverManager.chromedriver().setup();
driver = new ChromeDriver();
driver.get("http://www.programsbuzz.com/user/login");
driver.manage().window().maximize();
}
@Then("Enter the Username and Password")
public void Enter_the_Username_and_() {
driver.findElement(By.id("edit-name")).sendKeys("Naruto");
driver.findElement(By.id("edit-pass")).sendKeys("Uzu!221");
}
}
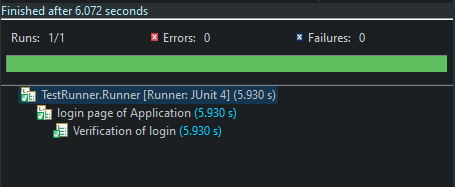
- Run the test from the runner class as a junit test.
- So we can see the test has run.
- This is how we can use the BDD framework with selenium.
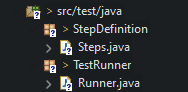
- Log in to post comments