We can use TestNG's Assertion or a simple if and else statement to verify the page title in Playwright using Java.
TestNG Assertion:
page.navigate("http://www.programsbuzz.com");
String title = page.title();
System.out.println(title);
Assert.assertTrue(title.contains("Technical"), "Match");
Using a simple assertTrue method which returns a boolean, we can assert the page title.
Assert.assertEquals(title, "ProgramsBuzz - Online Technical Courses");
We can also use the assert equals to pass in the expected and actual strings.
If Else:
page.navigate("http://www.programsbuzz.com");
String title = page.title();
String expectedTitle = "ProgramsBuzz - Online Technical Courses";
if (title.equalsIgnoreCase(expectedTitle)) {
System.out.println("Title Match Verfied");
} else {
System.out.println("Not a match!!");
}
- We can validate the page's title using a simple if else statement.
- we can see we stored both the expected and actual titles into the title and expectedTitle variable.
- We are using equalsIgnoreCase in the if statement we pass in both variables.
- If it matches, it goes inside if body.
- If it is not a match, it will execute the else body.
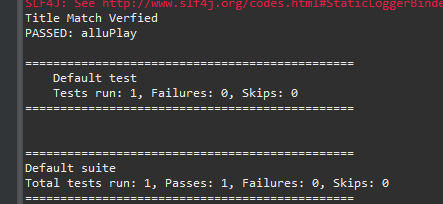
- Log in to post comments